Some cool tools used in, and developed for, the game
I’m a big fan efficiency. If there’s a task that involves mindless clicking for thirty minutes that I’m confident that I’ll be doing again at some point in the future, I’ll find a way to automate it or simplify it. Here are some of the tools I create for this project. Note that I’m a big fan of Odin Inspector and Serializer – this has cut my tool development time by probably 80% because I only have to spend time working on the logic, not the GUI…
Runtime Audio File Loading / Asset Swapping
For this project, I worked with an offsite contractor who produced all of the audio assets, and it was important for me to be able to provide for them a build of the game in which they could experiment with different sound effects and music. To achieve this, I have a few things going on, but it’s quite simple.
- Any object type that plays sound has an “Audio Settings” class nested inside; this has one or more audio clips serialized, along with a mixer group.
- A master “Game Audio Settings” class serializes an instance of each of these “Audio Settings” classes – this ends up looking a bit like this:
- Player
- Jump
- Land
- UI
- Interact
- Confirm
- Cancel
- Music
- Main Menu
- Level1
- GameOver
- Player
- To save the references to JSON, I use “Audio Clip Utility”’s ”Get Clip Names And Values” method, which takes any class and iterates over serialized fields (public or [SerializeField]) that are of the AudioClip type, then spits out a dictionary<string, string> with field names and clip names (this is the file serialized as JSON to StreamingAssets)
- At runtime, I use the same “Audio Clip Utility” class to load from the StreamingAssets folder, which updates all the references in the “Game Audio Settings” class
- Because all instantiated / pooled objects are injected with these settings, I don’t need to update anything in-game or in-editor
- Once the build is ready, any assets in StreamingAssets can be exchanged – if the file name changes, the changes can be reflected in the JSON file (in the same location), and when the game is run, the new clips are loaded.
This is a huge time saver for any audio artist who does not work in Unity or who just wants to hear their changes immediately.
Music Playback
This one’s super simple. There are three themes:
- Main Menu – Single track loop
- Game Over – Single track loop
- Gameplay – One track lead-up to single track loop
Prefab Search and Destroy
What happens when you’ve got a bunch of nested prefabs, and in each of them is an old or deprecated prefab that you want to get rid of? Enter this tool! Just select the nested prefab roots, select the offending prefab, and hit “Delete” – this tool does the rest.
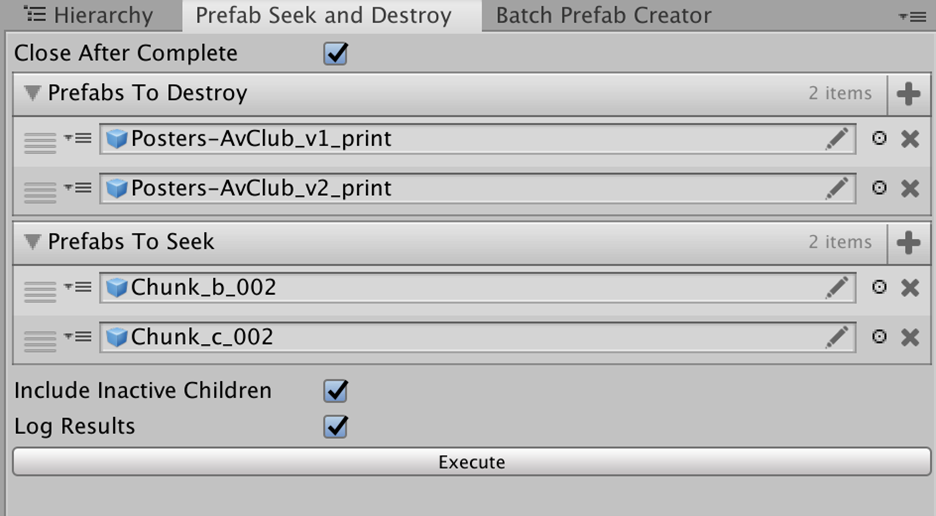
Create Multiple Sprites
When working in 2D, do you ever find yourself wanting to drag a bunch of sprites into the scene because they all serve a similar purpose, but for some reason Unity thinks you want to create an animation? Then this tool is for you! Simply select the sprites in Project view, then select Assets > Create > Multiple Sprites from Selection… from here, all the selected sprites now have their own game object in the scene view.
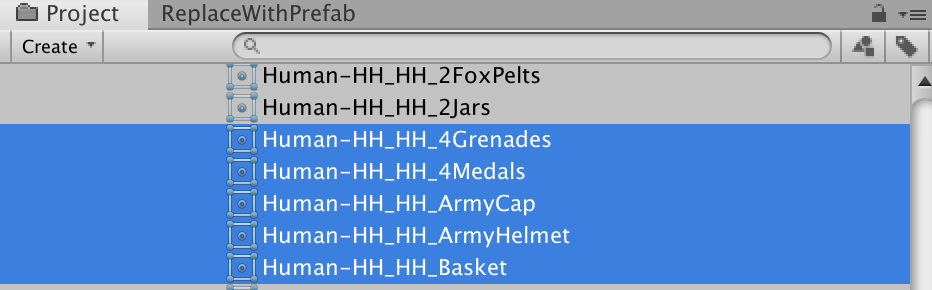
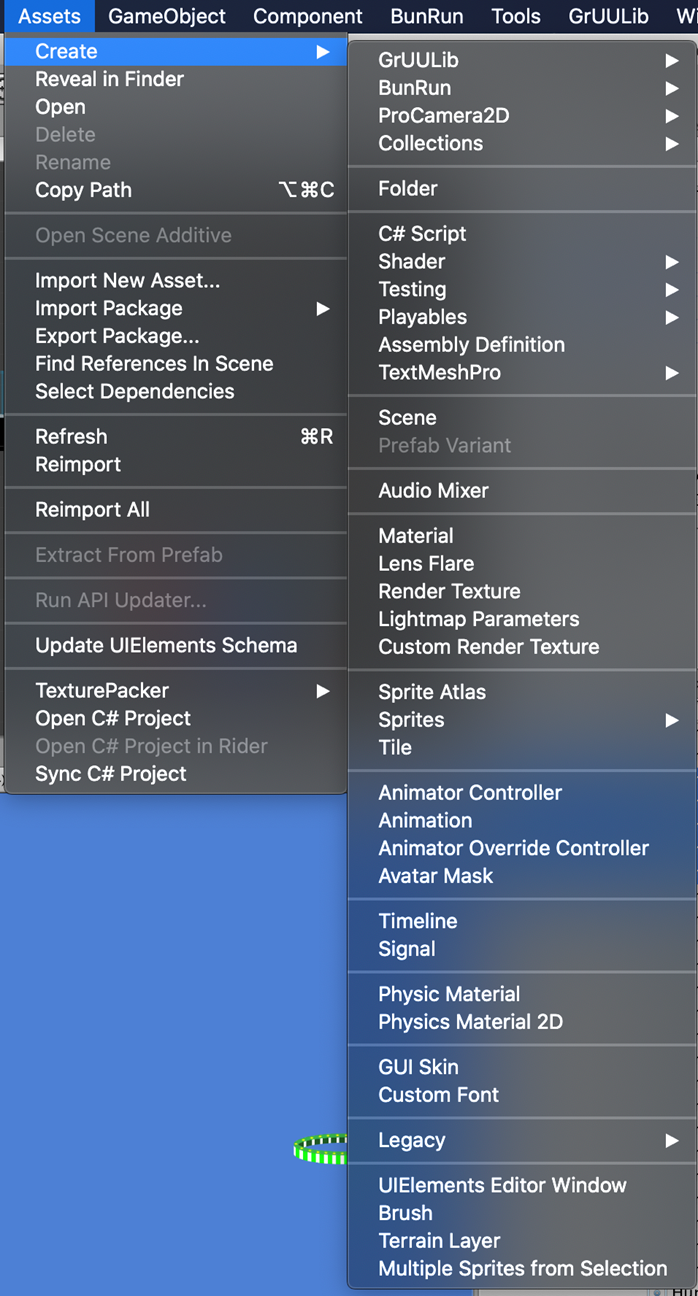
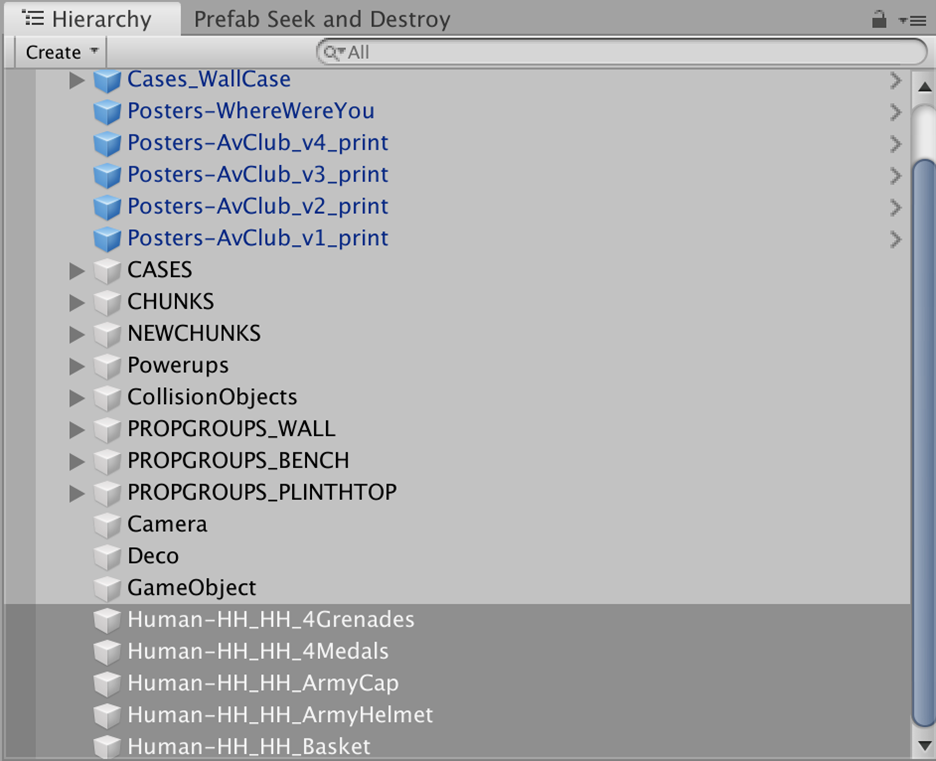
Batch Prefab Creator
This tool allows me to select a bunch of objects in the scene view, pop open the tool window, select a target directory, and hit “Create” – the result is a prefab has now been created in the specified directory for each selected object. You can even select which mode this follows, including Delete, Retain Original, and Replace.
Replace with Prefab
This is one that I didn’t create myself, but I’ll share the sources anyway because I use it all the time and it’s awesome.
https://unity3d.college/2017/09/07/replace-gameobjects-or-prefabs-with-another-prefab/ PS: I’m a really big fan of Jason Weimann of unity3d.college, from whom I’ve picked up most of my programming and development habits.
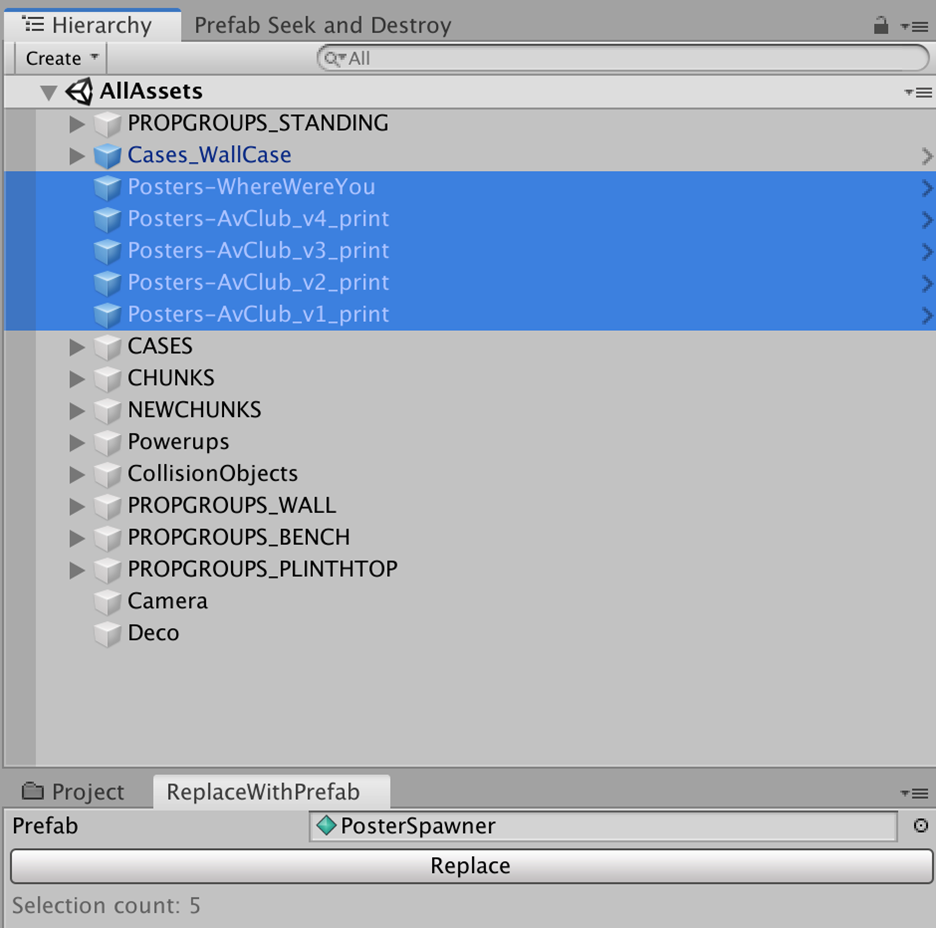
Moe's Mammoth Rampage
Dart through the museum collecting artifacts - watch out for bottomless pits!
Status | Released |
Author | RoyalAlbertaMuseum |
Genre | Platformer |
Accessibility | One button, Textless |
More posts
- Some technical details about the game!Oct 06, 2022